Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial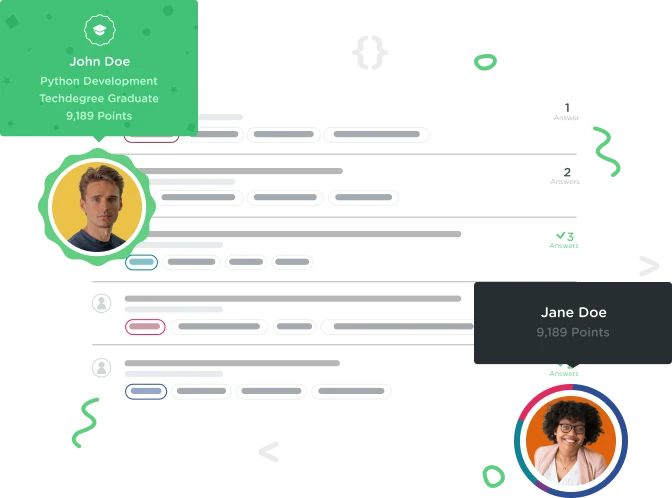

Radu Branis
Courses Plus Student 2,163 PointsBummer! Didn't get a 200 at /multiply
What is wrong with my code?
from flask import Flask
app = Flask(name)
@app.route('/multiply/5/5/') def multiply(num1, num2): return '{} * {} = {}'.format(num1, num2, num1 * num2)
from flask import Flask
app = Flask(__name__)
@app.route('/multiply/5/5/')
def multiply(num1, num2):
return '{} * {} = {}'.format(num1, num2, num1 * num2)
7 Answers

Dan Johnson
40,533 PointsYou'll want to have a new route expecting two variables rather than them being hard coded in.
You can do that like this:
@app.route("/multiply")
@app.route("/multiply/<num1>/<num2>")
def multiply(num1=5, num2=5):
#Not updated until later in the challenge.
return str(5 * 5)

james white
78,399 PointsI believe what Kenneth is saying is that this code will get you through up to Challenge 4 of 5:
from flask import Flask
app = Flask(__name__)
@app.route("/multiply")
@app.route("/multiply/<int:num1>/<int:num2>")
@app.route("/multiply/<float:num1>/<float:num2>")
@app.route("/multiply/<int:num1>/<float:num2>")
@app.route("/multiply/<float:num1>/<int:num2>")
def multiply(num1=5, num2=5):
#Not updated until later in the challenge.
return str(5 * 5)
However, then for Challenge 5 of 5 the return has to updated:
from flask import Flask
app = Flask(__name__)
@app.route("/multiply")
@app.route("/multiply/<int:num1>/<int:num2>")
@app.route("/multiply/<float:num1>/<float:num2>")
@app.route("/multiply/<int:num1>/<float:num2>")
@app.route("/multiply/<float:num1>/<int:num2>")
def multiply(num1=5, num2=5):
return str(num1 * num2)
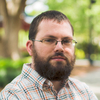
Kenneth Love
Treehouse Guest TeacherAlso, the view just wants back the result of 5 * 5
, not the mathematical sentence.

Avik Chakraborty
6,621 PointsStep1 from flask import Flask
app = Flask(name)
@app.route('/') @app.route('/multiply')
def multiply(): return str(5*5)
Lisa Sparks
3,187 PointsStill having problems, keep getting my code takes to long or Didn't get a 200 at multiply
from flask import Flask
from flask import request
app = Flask (__name__)
@app.route('/')
def index (name="Treehouse"):
name = request.args.get('name',name)
return "Hello from {}".format (name)
app.run(debug=True, port=8000,host='0.0.0.0')
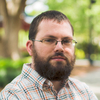
Kenneth Love
Treehouse Guest TeacherTake out the app.run()
. Someone else reported to me that that caused challenges to time out.
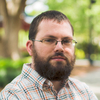
Kenneth Love
Treehouse Guest TeacherWell, you also didn't create a route at /multiply
like the instructions tell you to:
Add a view named
multiply
. Givemultiply
a route named/multiply
. Makemultiply()
return the product of5 * 5
. Remember, views have to return strings.
Lisa Sparks
3,187 PointsHaving problems with this problem
from flask import Flask
from flask import request
app=flask(__name__)
@app.route('1')
def index (name="Treehouse"):
return"Hello from {}". format(name)
name=request,args.get(name,name)
app,run(debug=True,port=8,000,host='0.0.0.0'
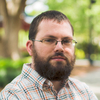
Kenneth Love
Treehouse Guest TeacherSo, a few problems.
- You want to use the
Flask
class that you imported for yourapp
variable.app = Flask(__name__)
- Your route should start with a
/
- You have a lot of spacing issues. Don't put spaces before or after dots, before or after parentheses, and don't leave spaces out after keywords like
return
. - You won't want to have your
return
before you do variable assignment and you'll want the variable assignment (the line starting withname=
) to be indented inside your function. - You want
app.run
notapp,run
. - You don't need a comma inside
8000
when it's the number.
Lisa Sparks
3,187 Pointsthanks Kenneth completed that challenge