Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial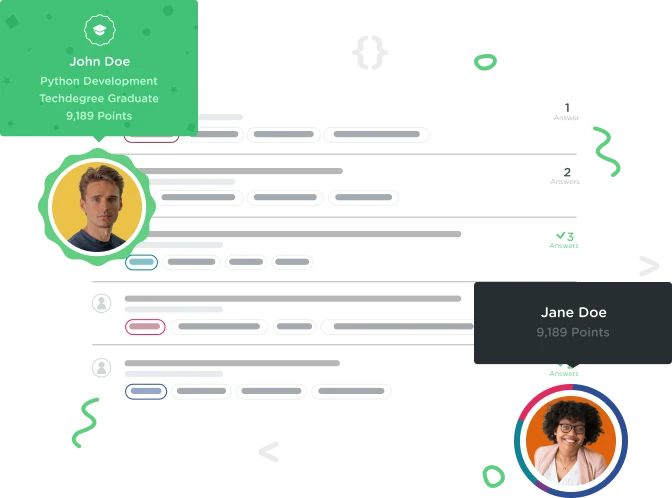

Ranish Malhan
Courses Plus Student 377 Pointscustom metabox data is not saving
<?php
/**
* Adds a box to the main column on the Post and Page edit screens.
*/
function offer_add_meta_box() {
$screen = 'offers';
add_meta_box(
'offer_meta',//section id
__( 'Offer Details' ),//section heading
'offer_meta_box_callback',// call callback function
$screen//post or page to show
);//end of add meta box function
}
add_action( 'add_meta_boxes', 'offer_add_meta_box' );//adding to action meta box
/**
* Prints the box content.
*
* @param WP_Post $post The object for the current post/page.
*/
function offer_meta_box_callback( $post ) {
// Add an nonce field so we can check for it later.
wp_nonce_field( 'offer_meta_box', 'offer_meta_box_nonce' );
/*
* Use get_post_meta() to retrieve an existing value
* from the database and use the value for the form.
*/
$value = get_post_meta( $post->ID, '_my_meta_value_key', true );
echo '<label for="offer_details">';
_e( 'Offer Details' );
echo '</label> ';
echo '<textarea id="offer_details" name="offer_details" value="' . esc_attr( $value ) . '" size="25"></textarea>';
}
/**
* When the post is saved, saves our custom data.
*
* @param int $post_id The ID of the post being saved.
*/
function offer_save_meta_box_data( $post_id ) {
/*
* We need to verify this came from our screen and with proper authorization,
* because the save_post action can be triggered at other times.
*/
// Check if our nonce is set.
if ( ! isset( $_POST['offer_meta_box_nonce'] ) ) {
return;
}
// Verify that the nonce is valid.
if ( ! wp_verify_nonce( $_POST['offer_meta_box_nonce'], '_meta_box' ) ) {
return;
}
// If this is an autosave, our form has not been submitted, so we don't want to do anything.
if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE ) {
return;
}
// Check the user's permissions.
if ( isset( $_POST['post_type'] ) && 'offers' == $_POST['post_type'] ) {
if ( ! current_user_can( 'edit_post', $post_id ) ) {
return;
}
}
/* OK, it's safe for us to save the data now. */
// Make sure that it is set.
if ( ! isset( $_POST['offer_details'] ) ) {
return;
}
// Sanitize user input.
$my_data = sanitize_text_field( $_POST['offer_details'] );
// Update the meta field in the database.
update_post_meta( $post_id, '_my_meta_value_key', $my_data );
}
add_action( 'save_post', 'offer_save_meta_box_data' );
1 Answer

Chris Shaw
26,676 PointsHi Ranish Malhan,
You appear to have a typo in your nonce verification which is the name your checking your $_POST
value again isn't correct, you currently have _meta_box when it should be offer_meta_box_nonce.
wp_verify_nonce( $_POST['offer_meta_box_nonce'], 'offer_meta_box_nonce' )
Hope that helps.
Ranish Malhan
Courses Plus Student 377 PointsRanish Malhan
Courses Plus Student 377 Pointsthanx