Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial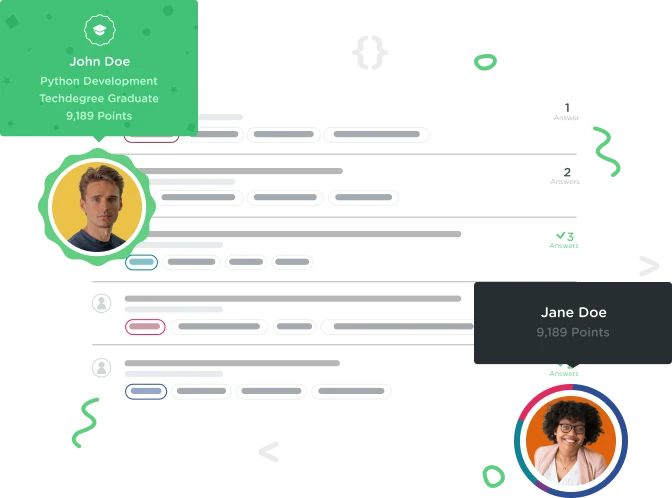
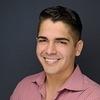
ian izaguirre
3,220 PointsHow do I tell Javascript to run a string only on a certain page?
Hi, so for example if I created a string that is a pop up prompt that says "what is your name" , How do I make it so this pop up prompt only appears on the contact me page and the terms of use page for example on my wordpress website ?
How do you specify what pages you want certain strings to run on ?
2 Answers
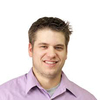
Kevin Korte
28,149 PointsWordpress gives you a is_page
function.
So for instance you can put your JS alert inside of that function, so it only gets included if that function evaluates to true, so for instance:
<?php
is_page( 'contact') || is_page( 'terms-of-use' ) {
?>
<script>
alert( "What is your name?" );
</script>
<?php
}
?>
What you put inside the is_page function depends on the page. It can be the page title, page ID, or page slug. That bit of JS will only be sent to the browser by the server, if that PHP block evaluates true.
Obviously, you can put whatever you want inside of the script tags for JS. I just threw in a cheesy alert for demo purposes.
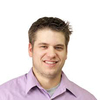
Kevin Korte
28,149 PointsLet me break some things down further. When I say I'm using the server to control what gets sent to the browser, what I mean is I'm doing it in PHP, since PHP is a server side language. Simple as that. Wordpress is PHP, and basically Wordpress does the same thing, it uses PHP to determine what posts, widgets, etc get sent to the browser.
The easiest way to do this is going to be PHP, since Wordpress gives you some pre-built functions you can just use, instead of having to create your own.
Take a look at this documentation: http://codex.wordpress.org/Function_Reference/is_page
If you had two WP pages named "Contact" and "Terms of Use", you could use those as the argument to check with Wordpress's is_page function. All of this is going back to the first bit of code I wrote.
You could put that block of code in either the function.php file, or just put it on the pages you want. Which than kind of negates the need to check for that page since you manually put it there.
ian izaguirre
3,220 Pointsian izaguirre
3,220 Pointshi thanks for helping me I don't understand though why you have php and script tags written? Is this not all done solely in the js file im working in?
Kevin Korte
28,149 PointsKevin Korte
28,149 PointsNo, at least not the way I'm writing. I'm using the server to control what does and does not get sent up the the client's browser. If the server evaluates that PHP statement as true, you can send whatever you want inside of that block to the browser.
You can't put JS inside a PHP tag, so I have to close the PHP tag, do my JS thing, and than reopen a PHP tag to finish off my PHP if statement. The server will send whatever is inside that if statement even though it is in 2 different PHP tags, it's doesn't care.
If you wanted your JS to be in a separate JS file, you could just include that file as the script source, and you'll have the same result.
IMO, this is better than checking the page with JS. It should be faster. If you wanted to do it in JS, you'll have to write an if loop in JS first to check for some unique identifier like a tittle or something that this is the page you want the script to run on.
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsHi , your saying your using the server to control what gets sent, I do not understand this as I am only working with wordpress , it would make more sense to me if you would say you write this code in index.php file of your theme etc... lol I am just learning so I do not know what location to go to for your first method of php.
When you said : "If you wanted to do it in JS, you'll have to write an if loop in JS first to check for some unique identifier like a tittle or something that this is the page you want the script to run on."
This makes more sense to me, even though its still a little confusing. So your saying I can use and If/Else statement to tell a string to activate on a specific page ? so for example for a terms of use page where that is the only location on the website where the words terms of use are used then would it be something like this ? :
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsAny updates ? :-)