Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial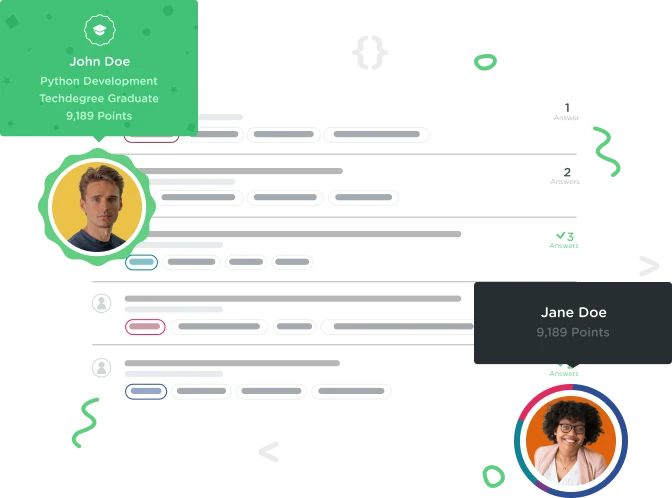

Deven Schmidt
7,847 PointsHow does cards[0] and cards[1] represent matched cards?
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
When printing the self.cards words the first two words are "add" how is this being used to match the cards?
[MOD: added ```python formatting -cf]
2 Answers
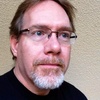
Chris Freeman
Treehouse Moderator 68,441 PointsHey Deven Schmidt, this an excellent question! The answer is found by following how Python passes object references.
The cards[0]
and cards[1]
hold references to specific cards within the self.cards
list.
I’ve added comments and two print statements to show the card ids. If ids match then the objects are the same object.
def check_match(self, loc1, loc2):
print(f”self.card ids: {[id(x) for x in self.cards]}”)
cards = []
# self.cards contains all the cards
for card in self.cards:
# card references individual cards in self.cards
if card.location == loc1 or card.location == loc2:
# card appended references a specific card in self.cards
cards.append(card)
if cards[0] == cards[1]:
# the objects in cards list are references to specific cards in self.cards
# set the match attribute on these specific cards if the card attribute as seen by the __eq__ method match
print(f”card[0] is: {id(card[0])}:{card[0].card}, card[1] is: {id(card[1])}:{card[1].card}”)
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
Edit: show explicit word value in each card
Post back if you need more help. Good luck!!!

Deven Schmidt
7,847 PointsThank you so much, struggled over that idea for a bit!
Asher Orr
Python Development Techdegree Graduate 9,409 PointsAsher Orr
Python Development Techdegree Graduate 9,409 PointsHey Chris, thanks for this response!
I am still confused about this.
Here's my line of thinking:
I see that the objects in the cards list are references to specific cards in self.cards. However, the first card (cards[0]) and the second card being compared (cards[1]) are referencing two separate cards within the self.cards list. I don't see how Python would return "True" for the statement:
if cards[0] == cards[1]:
Any idea on where I'm going wrong here?
Thanks again!
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsHey Asher Orr! That’s a very good question. First let me state that the overuse of “card” in this code can be confusing.
Looking back at the Card class video, the
Card
class defines the comparison method__eq__
to returnTrue
if theCard.card
(ugh!) attributes of twoCard
instances are the same. That is, if the word stored in the twocards
are the same. These two lines would be equivalent:I’ll need to edit my answer above to clarify this. Thanks!
Post back if you need more help. Good luck!!!
Asher Orr
Python Development Techdegree Graduate 9,409 PointsAsher Orr
Python Development Techdegree Graduate 9,409 PointsThat makes perfect sense! Thanks so much for your response!