Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial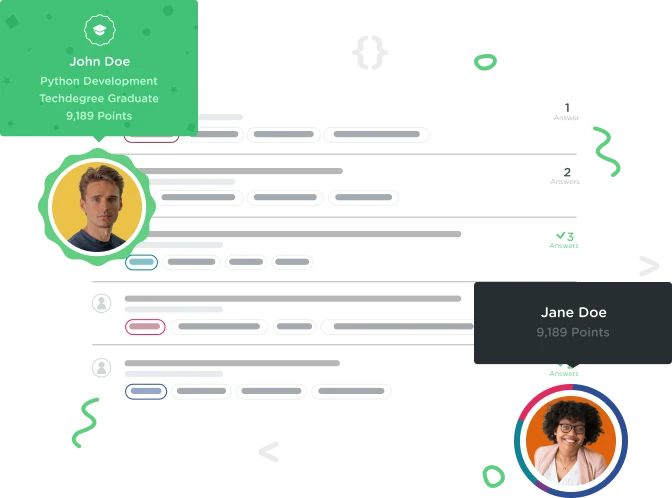

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 Points__init__ question
The question states: Our Student class is coming along nicely! I'd like to be able to set the name attribute at the same time that I create an instance. Can you add the code for doing that? Remember, you'll need to override the init method.
I'm stuck on how to do this. I tried googling how to override the init method and the sample code below was something close to what other people were saying in stackoverflow but obviously this is not correct.
class Student:
name = "Your Name"
def __init__(self, name=None, **kwargs):
super(Student,self).__init__()
self["name"]=name
for key, value in kwargs.items()
setattr(self,key,value)
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
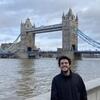
Gonzalo Mordecki
13,746 PointsHi! I think you are overthinking it!
The first challenge, you just have to do self.name = name, so when the class is instanced, name is going to be a requirement:
class Student:
def __init__(self, name):
self.name = name
The second one requires to use setattr
:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
8 Answers

Kent Åsvang
18,823 PointsOkay, so you are pretty close, I have added some comments that I hope will help you understand what is going on:
""" You don't have to initiate name with a value, the argument alone is enough """
def __init__(self, name=None, **kwargs):
""" super() - calls methods on the parent class, and is not necessary in this challenge."""
super(Student,self).__init__()
"""this line is fine, although I prefer dot-notation myself. 'self.name'
self["name"]=name
""" This one is also correct, just remember to put the colon after 'items():'"""
for key, value in kwargs.items()
setattr(self,key,value)
Here is my solution :
def __init__ (self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
Hope this was helpful.

Mercedes Aker
6,544 PointsThis I have also tried but it continues to say, "Try again!"

Tobert Nasibu Amulani
8,423 Pointsstill its not working out

goodness mate
93 Pointsclass Student: name = "Your Name"
def __init__(self, grade):
if grade>50:
return self.praise
else:
return self.reassuran

Joseph Doma
3,055 Pointsplease help me on the int thing

CHO Ming TSENG
14,219 Pointsclass Student: def init(self,name): self.name=name

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsWell that's annoying.
My first attempt had everything you had, but I just forgot the ":" sign after the for statement.
Thanks!

Kent Åsvang
18,823 PointsIt's very typical to forget small stuff like that. I would recommend using pycharm, or a text-editor on the side while walking through the tracks here on treehouse. That way you can get used to the error-messages and to find bugs like this in your code

Michael Ma
4,294 Pointsclass Student: def init(self, name): self.name = name
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
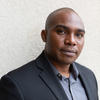
pierreilyamukuru
9,831 Pointsclass Student: name = "Pierre" def init (self, name, **kwargs): self.name = name for key, value in kwargs.items(): setattr(self, key, value)
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
Godfrey Chamunorwa
5,505 PointsGodfrey Chamunorwa
5,505 Pointsyou remove the super class its not necessary and after that line its self.name = name put the colon : after the items(): then you will get it write