Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial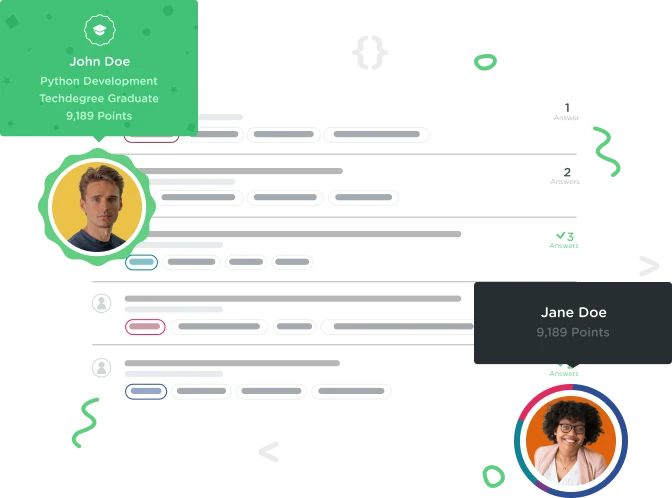
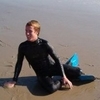
Jeremy Frimond
14,470 PointsNumbered Pagination on Custom Post Types and Regular Post Type
I am looking to make a numbered pagination bar at the bottom of some pages containing lots of posts. Is there a video or article or any other recommendation type on how to get this working on a custom built WP theme?
Cheers
6 Answers

Ryan Duchene
Courses Plus Student 46,022 PointsThere are a couple WordPress functions you can use that get you most of the way there, and the rest is just some simple math. Here's a basic pagination template part:
<?php
/**
* Total number of published posts in the database that are viewable by everyone
*
* @var int
**/
$posts_count = wp_count_posts()->publish;
/**
* Maximum number of posts in a listing page
*
* @var int
**/
$posts_per_page = get_option( 'posts_per_page' );
/**
* Number of pages required to display all the posts ALL THE POSTS
*
* @var int
**/
$pages_of_posts = (int) ceil( $posts_count / $posts_per_page ); // because division returns floats, cast to int
/**
* Current page of posts
*
* @var int
**/
$paged = ( get_query_var( 'paged' ) ? get_query_var( 'paged' ) : 1 );
?>
<div class="posts-nav">
<ul class="c posts-nav-c">
<?php if ( $paged !== 1 ): // generate the a link to the first page if we're not already on it ?>
<li class="posts-nav-newest">
<a href="<?php echo $GLOBALS['PHP_SELF']; ?>">
1
</a>
</li>
<?php endif; ?>
<?php if ( ( $newer = $paged - 2 ) > 1 ): // generate a link to two pages back if it exists ?>
<li class="posts-nav-newer-2">
<a href="<?php echo $GLOBALS['PHP_SELF'] . '/page/' . $newer . '/'; ?>">
<?php echo $newer; ?>
</a>
</li>
<?php endif; ?>
<?php if ( ( $newer = $paged - 1 ) > 1 ): // generate a link to one page back if it exists ?>
<li class="posts-nav-newer">
<a href="<?php echo $GLOBALS['PHP_SELF'] . '/page/' . $newer . '/'; ?>">
<?php echo $newer; ?>
</a>
</li>
<?php endif; ?>
<li class="posts-nav-current">
<?php echo $paged; // a holder for the current page ?>
</li>
<?php if ( ( $older = $paged + 1 ) < $pages_of_posts ): // generate a link to one page ahead if it exists ?>
<li class="posts-nav-older">
<a href="<?php echo $GLOBALS['PHP_SELF'] . '/page/' . $older . '/'; ?>">
<?php echo $older; ?>
</a>
</li>
<?php endif; ?>
<?php if ( ( $older = $paged + 2 ) < $pages_of_posts ): // generate a link to two pages ahead if it exists ?>
<li class="posts-nav-older-2">
<a href="<?php echo $GLOBALS['PHP_SELF'] . '/page/' . $older . '/'; ?>">
<?php echo $older; ?>
</a>
</li>
<?php endif; ?>
<?php if ( $paged !== $pages_of_posts ): // generate a link to the last page if we're not already on it ?>
<li class="posts-nav-oldest">
<a href="<?php echo $GLOBALS['PHP_SELF'] . '/page/' . $pages_of_posts . '/'; ?>">
<?php echo $pages_of_posts; ?>
</a>
</li>
<?php endif; ?>
</ul>
</div>
This could be tweaked to be a little more intelligent; for instance, I could allow administrators to view private/protected posts. But it works well enough for me.
Hope this helps!
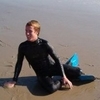
Jeremy Frimond
14,470 PointsJames Callahan I figured it out- took lots of head banging against wall hours! I will write something out and post it here once I have it all loaded to my site. Hopefully it can help you out
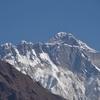
Bob Sutherton
20,160 PointsOh, that's great! Thanks, I would appreciate being able to see what you came up with.

Zac Gordon
Treehouse Guest TeacherPlease do!
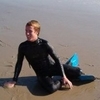
Jeremy Frimond
14,470 PointsRyan Duchene thanks for the snippet, I am looking into this now. I have some basic "silly" questions to ask:
Do I need to change any of the code to fit my project? such as post per page?
What file and where within that file would I put this code?
cheers

Ryan Duchene
Courses Plus Student 46,022 PointsNah, those aren't silly at all! :) Sorry about not getting back fast; I had to be somewhere.
I'm highly confident that it works across any WordPress install, but haven't done the testing to prove it. The $posts_per_page
variable uses a little trick with WordPress where it uses a built-in option (changeable by the end user) that's in the WP admin area, so that should work globally as well, with maybe a (very) rare exception if someone uses a heavily customized WordPress install.
I know for certain that you can put it in your home.php
file (list of blog posts) and it'll work. I think it'll work as-is for custom post types, but I'm not a hundred.
My recommendation would be to put it in a template part (maybe interface-pagination.php
) and then include it with this function:
<?php
get_template_part( 'interface', 'pagination' );
If you have any more questions, feel free to ask me!
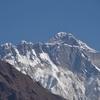
Bob Sutherton
20,160 PointsThanks Ryan. I may put some questions here as well.
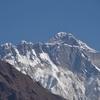
Bob Sutherton
20,160 PointsI imagine this is a question that a lot of us have. It would be cool if Zac Gordon did a workshop on how to set this up. The above is just a bunch of code and your "silly" questions are probably no match for my extra-stoopid questions! Again, it is just one long block of code. Not that I don't appreciate Ryan taking the time to post it.
My first theory is that I should print that code block out on paper and throw it at the computer screen, thereby creating a mini-cosmic collision that will result in pagination!
Just having a little fun. Jeremy, I have also been having some difficulty getting pagination. I have been looking at the WordPress codex but it definitely "ain't writ for dummies" like me. I still don't have a good enough grasp of looping and PHP yet I think.
I wonder if there are any PLUGINS that someone could recommend to make this a more basic operation?

Zac Gordon
Treehouse Guest TeacherHi folks! I'll see what I can do to roll in a discussion of custom pagination somewhere in a course.. there are number of plugins that do this functionality for you too if you don't want to code it yourself :)
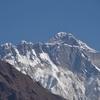
Bob Sutherton
20,160 PointsI was so dumb that I didn't even know that you could use wordpress' default pagination on individual posts by doing this:
Place this in the HTML version of the post. <!--nextpage-->
Here is the video where I learned how to do it!
Of course, I am still trying to work out how to paginate my "Articles" custom post type that I have displayed in a loop. The loop lists out the name of the article, an image associated with it, and an excerpt from the article. I need to paginate this page so that it is not one loooong list of article excerpts.
Bob Sutherton
20,160 PointsBob Sutherton
20,160 PointsI tried using this with the get_template_part method you recommended a little further down the page. I didn't have any success.
A bulleted number "one" did appear at the bottom of the page, which made me feel like it was close. But no cigar.
Any thoughts?