Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial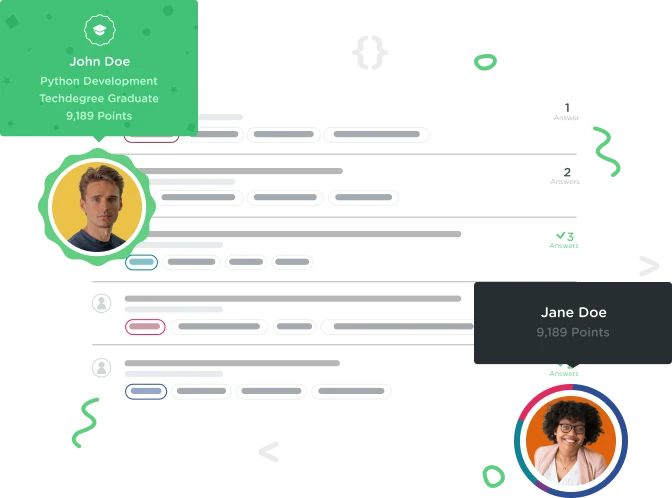

Tyler Zika
14,402 PointsRepeat jQuery in Wordpress loop
I've used jQuery to implement a hide/show button for the comments of each post on a Wordpress blog http://zealouslyzika.com.
If you notice, the hide/show button only works on the first post on the front page, and on each individual post when a user clicks on the post link.
How do I make the hide/show button repeat on each post so the comments are always able to shown/hidden at the users discretion?
Here is jQuery code that I placed in the footer.php file.
<script>
// Show/Hide Comments
jQuery(document).ready(function() {
// Get #comment-section div
var commentsDiv = jQuery('#single_post_comments'); <-- This is the name of the div id wrapped around the WP php comment loop code
// Only do this work if that div isn't empty
if (commentsDiv.length) {
// Hide #comment-section div by default
jQuery(commentsDiv).hide();
// Append a link to show/hide
jQuery('<button/>')
.attr('class', 'toggle-comments')
.attr('href', '#')
.html('Show Comments ')
.insertBefore(commentsDiv);
// Encase button in #toggle-comments-container div
jQuery('.toggle-comments').wrap(jQuery('<div/>', {
id: 'toggle-comments-container'
}))
// When show/hide is clicked
jQuery('.toggle-comments').on('click', function(e) {
e.preventDefault();
// Show/hide the div using jQuery's toggle()
jQuery(commentsDiv).toggle('slow', function() {
// change the text of the anchor
var anchor = jQuery('.toggle-comments');
var anchorText = anchor.html() == 'Show Comments ' ? 'Hide Comments ' : 'Show Comments ';
jQuery(anchor).html(anchorText);
});
});
} // End of commentsDiv.length
}); // End of Show/Hide Comments
</script
3 Answers

Zac Gordon
Treehouse Guest TeacherYou'll need to write your JS in a way that uses this and parents and children so that it is relatively referencing the content you want to show and hide.
Here is the basic logic I would use:
1. Use JS to hide all the comment fields
2. Use JS to add the show hide button in the right place
3. On click of the button go to the closest comment (next sibling?) and toggle the state
Hopefully that helps!

Zac Gordon
Treehouse Guest TeacherPS - awesome site! Digging that super rainbow pic :)
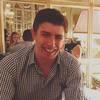
Matt Campbell
9,767 PointsHi Tyler Zika This might help you as it does something vaguely similar. Basically, there's a div that's 200% the width of the viewport. Left side has a list of post titles, right side has the content of the posts, all stacked atop of one another.
Idea is, being a mobile app, user hits which post (it's an exercise app so it's the title of the workout), and across slides the information for how many reps to do, weight to use and video on how to complete the exercise.
That's all not so relevant but gives context. However, what is relevant is that the jQuery works on each title, similar to your button, for each post title in the list. Getting, sliding over the content relative to that title.
There's a count in the loop that generates the id number for each title so that the corresponding content is slid over. To go back, the parent of the button needs to be moved so we're using .parent().
As I said, I don't know if that will help but hope it does a bit.
<script>
$('.left-list-item').click(
function(){
var listId = $(this).attr('id');
//console.log(listId);
$('#right-list-item-' + listId).addClass('over').animate({left:"-=100%"}, 500);
//console.log('.right-list #' + listId);
});
$('.right-list-back').click(
function(){
var backId = $(this).parent().attr('id');
//console.log(backId);
$('#' + backId).removeClass('over').animate({left:"+=100%"}, 500);
//console.log(backId);
});
</script>